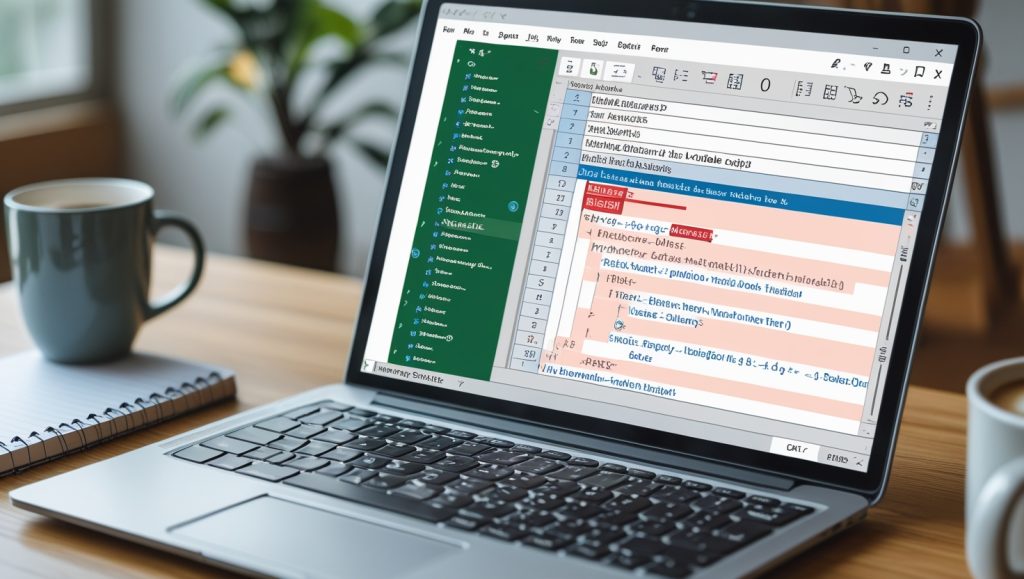
ver stared at a cryptic error message in Excel VBA, feeling like your spreadsheet dreams are crumbling? Don’t panic! Mastering how to debug and fix errors in Excel VBA can turn frustration into triumph—and I’m here to show you how. Stick with me, and by the end of this article, you’ll have a toolbox of practical techniques to squash those bugs like a pro. Ready to take control? Let’s dive in!
Introduction
Excel VBA (Visual Basic for Applications) is a powerful tool for automating tasks, building custom functions, and streamlining workflows. However, even the savviest coders hit roadblocks—runtime errors, syntax slip-ups, or logic glitches that grind your macros to a halt. Fortunately, learning to debug and fix errors in Excel VBA doesn’t have to be a nightmare. In this guide, I’ll walk you through step-by-step methods to identify, troubleshoot, and resolve common VBA issues. Whether you’re a beginner or a seasoned user, these tips will save you time and headaches. Let’s get cracking!
Why You Need to Debug and Fix Errors in Excel VBA
Before we jump into the how-to, let’s understand the why. Errors in VBA can derail your projects—think incorrect calculations, crashed spreadsheets, or hours of wasted effort. By mastering debugging, you ensure your code runs smoothly, delivering reliable results. Plus, it’s a skill that boosts your confidence and efficiency. Ready to tackle those pesky bugs? Here’s how to debug and fix errors in Excel VBA like an expert.
1. Understand Common VBA Error Types
First, let’s identify the enemy. VBA errors fall into three main categories:
- Syntax Errors: Typos or incorrect commands (e.g., missing parentheses).
- Runtime Errors: Issues that pop up while the code runs (e.g., “Run-time error ‘1004’”).
- Logic Errors: The code runs but produces wrong results (e.g., a loop that skips rows).
Knowing these helps you pinpoint the problem faster. For instance, a syntax error is easy to spot in the editor, while runtime errors require deeper digging.
2. Use the VBA Editor’s Built-In Tools
The VBA Editor (accessed via Alt + F11) is your debugging HQ. Start with these features:
- Immediate Window: Press Ctrl + G, type ?variableName, and hit Enter to check values.
- Locals Window: View all active variables and their states during execution.
- Watch Window: Monitor specific variables for changes.
These tools let you peek inside your code’s brain, making it easier to debug and fix errors in Excel VBA.
3. Step Through Your Code with F8
Next, slow things down. Hit F8 in the VBA Editor to execute your code line by line. This “Step Into” feature reveals exactly where things go wrong. For example, if a runtime error crashes your macro, stepping through shows which line trips it up. Paired with the Locals Window, this method is gold for spotting issues in real-time.
4. Add Breakpoints to Pause Execution
Sometimes, you suspect a specific section is the culprit. Click the gray margin left of a line to set a breakpoint (a red dot appears). Run your code with F5, and it’ll pause at that spot. From there, inspect variables or step through to see what’s misfiring. Breakpoints are a fast track to debug and fix errors in Excel VBA without wading through every line.
5. Leverage MsgBox for Quick Checks
Want a simple way to test values mid-code? Insert a MsgBox statement like this:
MsgBox "Value of x is: " & x
Run the macro, and a pop-up displays the variable’s value. It’s a low-tech but effective trick to confirm your code’s behavior. If the output surprises you, you’ve found a clue to the error!
6. Handle Errors with On Error Statements
Prevention is better than cure. Use On Error to manage runtime issues gracefully:
On Error Resume Next ‘Skips the error and moves on
‘Your code here
On Error GoTo 0 ‘Resets error handling
Alternatively, redirect to a custom error handler:
On Error GoTo ErrHandler
‘Your code here
Exit Sub
ErrHandler:
MsgBox "Oops! Error: " & Err.Description
This approach keeps your macro from crashing while you debug and fix errors in Excel VBA.
7. Check for Common Culprits
Many VBA errors stem from predictable slip-ups. Watch out for:
- Uninitialized Variables: Always declare and set values (e.g., Dim x As Integer: x = 0).
- Object References: Ensure objects like worksheets exist (e.g., Set ws = ThisWorkbook.Sheets(“Sheet1”)).
- Loop Issues: Verify your For or Do loops terminate correctly.
Spotting these early saves you tons of debugging time.
8. Use Debug.Print for a Paper Trail
Another handy trick is Debug.Print. Add it to your code like this:
Debug.Print "Step 1: " & Range("A1").Value
Check the Immediate Window (Ctrl + G) to see the output. It’s like leaving breadcrumbs to trace your code’s path—perfect for logic errors that don’t crash but still mess up results.
9. Test Small Code Chunks
Big macros can feel overwhelming. Break them into smaller pieces and test each part. For instance, if your code formats a table then emails it, test the formatting alone first. Isolating sections helps you debug and fix errors in Excel VBA without drowning in complexity.
10. Consult Online Resources and Communities
Finally, don’t go it alone. Stuck on a stubborn error? Search forums like Stack Overflow or Microsoft’s VBA documentation. Use specific keywords (e.g., “Excel VBA runtime error 13”) to find solutions fast. The VBA community is full of pros who’ve tackled the same bugs you’re facing!
Bonus Tips to Master Debugging
Here’s how to level up your skills:
- Comment Your Code: Add notes (e.g., ‘This loop counts rows) to track your logic.
- Save Often: Avoid losing work when Excel crashes mid-debug.
- Practice: Write small test scripts to hone your troubleshooting chops.
With these habits, you’ll debug like a seasoned coder in no time.
Conclusion
There you have it—a complete playbook to debug and fix errors in Excel VBA. From stepping through code to handling errors like a pro, these 10 techniques will transform you from a VBA victim to a debugging victor. Start applying them today, and watch your macros run smoother than ever. Which tip are you trying first? Drop a comment below—I’d love to hear your success stories! Keep exploring Insightsica for more tech tricks to boost your skills. Happy coding!
Also, Follow us @insightsica_ai on Instagram and blog for more update